Go基础|第1章:Get started with Go
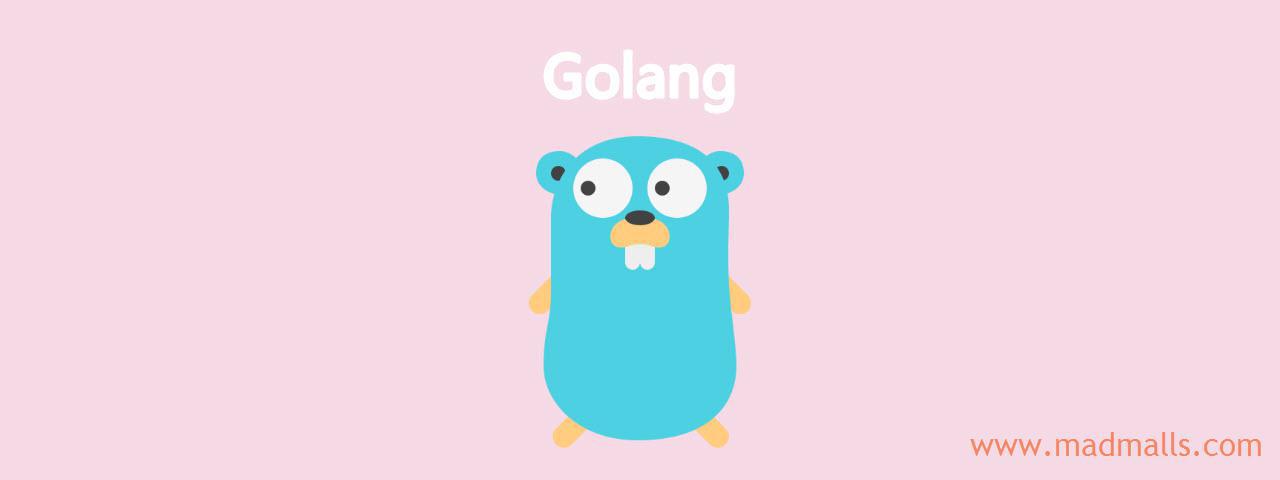
Synopsis: 工欲善其事,必先利其器。本文介绍如何在 Windows 或 Linux 系统中安装 Go,使用 Go Modules 模块管理器、并通过设置 GOPROXY 代理服务器来安装那些国内无法正常访问的第三方模块
1. 安装 Go
Go 项目开源在 Github,我们可以直接到 国际官网 或 国内官网 下载安装程序即可
1.1 Windows 安装 go1.15
如果你是 Win10 系统的话,下载 MSI 二进制程序包,比如 go1.15.2.windows-amd64.msi
。双击后运行,依次点击下一步即可(默认安装在 C:\Go\
位置)。安装完成后,会自动在 PATH
系统环境变量中追加 C:\Go\bin
,所以我们按 Ctrl + R
打开 CMD
命令行窗口后,可以直接运行 go
命令了,比如查看 Go 的版本:
Microsoft Windows [版本 10.0.14393] (c) 2016 Microsoft Corporation。保留所有权利。 C:\Users\wangy>go version go version go1.15.2 windows/amd64
1.2 Linux 安装 go1.15
如果你想在 Linux 系统中安装 Go:
1. 下载与解压 # wget https://golang.google.cn/dl/go1.15.2.linux-amd64.tar.gz # tar -xzf go1.15.2.linux-amd64.tar.gz -C /usr/local 2. 设置环境变量 # echo -e "export PATH=$PATH:/usr/local/go/bin" >> /etc/profile # source /etc/profile 3. 验证 # go version
2. 熟悉 Go 相关子命令
安装完 Go 之后,可以通过 go
命令来使用官方提供的工具链(tool chain),实现对源代码进行 编译(go build)
、安装(go install)
、运行(go run)
、下载第三方包(go get)
等操作:
bug start a bug report build compile packages and dependencies clean remove object files doc show documentation for package or symbol 查看包文档 env print Go environment information 查看或修改 Go 环境变量 fix run go tool fix on packages fmt run gofmt on package sources 使用官方风格格式化 Go 代码 generate generate Go files by processing source get download and install packages and dependencies 使用 Git 等代码版本控制软件将一个远程 Go 代码库拉取到本地,以便将其做为第三方 Go 包引入并使用 install compile and install packages and dependencies list list packages mod module maintenance 维护 Go 模块 run compile and run Go program test test packages 运行单元测试和基准测试 tool run specified go tool version print Go version vet run go tool vet on packages 检查可能的代码逻辑错误
使用 go help env
查看子命令的详细使用文档,比如查看 Go 环境变量(相同名称的系统环境变量优先级更高):
C:\Users\wangy>go env ... set GOOS=windows set GOROOT=D:\Go1.15 set GOPATH=C:\Users\wangy\go set GOPROXY=https://proxy.golang.org,direct ...
设置:
取消设置:
更加详细的 go
命令使用方法,请参考: https://golang.google.cn/cmd/go/
3. 设置 Go 模块代理: GOPROXY
安装好 Go 之后环境变量 GOPROXY
的默认值为 https://proxy.golang.org
代理服务器地址,但是在国内无法访问,导致我们还是无法通过 GOPROXY 代理来安装 golang.org/x/*
包(比如 golang.org/x/sys
、golang.org/x/net
、golang.org/x/tour
等)
幸好,像 https://goproxy.cn
(由七牛云运行,支持 CDN 加速) 和 https://goproxy.io
这两个同样实现了 Module proxy protocol 协议的代理服务器在国内是能够访问的,所以我们只需要修改一下 GORPOXY
的值即可:
注意:如果你使用
Goland
IDE 进行代码编辑的话,还需要手动设置File
→Settins...
→Go
→Go Modules (vgo)
→Proxy
→https://goproxy.cn,direct
4. 编辑器插件和IDE
- vim:
vim-go
plugin provides Go programming language support - Visual Studio Code: Go extension provides support for the Go programming language
- GoLand: GoLand is distributed either as a standalone IDE or as a plugin for IntelliJ IDEA Ultimate
- Atom:
Go-Plus
is an Atom package that provides enhanced Go support
5. Hello World
使用 Goland 打开 D:\GoCodes\hello
目录,创建 hello.go
文件:
package main // 定义 main 包 import "fmt" // 导入标准库中的 fmt 包 func main() { // main 函数 fmt.Println("Hello, World!") // 调用 fmt 包中导出的 Println() 函数 }
Go 的标准库位于 %GOROOT%\src
目录中,比如 C:\Go\src
。所以,fmt
包的目录为 C:\Go\src\fmt\
然后在 CMD 中运行:
6. Go Modules
- 执行帮助命令:
go help modules
- https://golang.org/doc/tutorial/create-module
- https://golang.google.cn/doc/tutorial/create-module
修改上面的代码,调用外部第三方模块 rsc.io/quote
:
再次在 CMD 中运行:
D:\GoCodes\hello>go run hello.go hello.go:6:2: cannot find module providing package rsc.io/quote: working directory is not part of a module
如果你的代码需要导入第三方模块中的包时,需要将你的代码组织成 模块(module)
,然后借助 Go Modules 来自动管理依赖。Go 1.11 版本需要设置 GO111MODULE=on
环境变量来启用 Go Modules
,从 Go 1.13 版本开始,默认已经启用了 Go Modules
(GO111MODULE 的值为 auto 或空)
D:\GoCodes\hello>go mod init github.com/wangy8961/hello go: creating new go.mod: module github.com/wangy8961/hello
执行上述命令后,会在当前目录下生成 go.mod
文件,此时它的内容如下:
然后,你再执行 go run
或 go build
命令时,Go Modules
会自动帮你查找依赖并下载到本地(依赖的第三方模块被下载到 %GOPATH%\pkg\mod
目录中,比如 D:\GoCodes\pkg\mod
,默认下载依赖模块的最新版本),非常方便!
D:\GoCodes\hello>go run hello.go go: finding module for package rsc.io/quote go: found rsc.io/quote in rsc.io/quote v1.5.2 Don't communicate by sharing memory, share memory by communicating.
此时你再查看 go.mod
文件的内容:
require
关键字表示依赖 v1.5.2 版本的 rsc.io/quote 模块,go.mod 文件中还可能存在 replace
和 exclude
关键字,分别表示替换依赖模块和忽略依赖模块
使用 Go Modules 管理依赖模块时,还会创建 go.sum
文件,它的每一行的格式大致如下:
分别表示模块路径、模块版本号、以 h1: 开头的模块 Hash 值
如果远程模块使用了 tag 表示版本号时:
未使用版本号时:
golang.org/x/tools v0.0.0-20190524140312-2c0ae7006135/go.mod h1:RgjU9mgBXZiqYHBnxXauZ1Gv1EHHAz9KjViQ78xBX0Q=
go mod
的其它子命令:
D:\GoCodes\hello>go help mod Go mod provides access to operations on modules. Note that support for modules is built into all the go commands, not just 'go mod'. For example, day-to-day adding, removing, upgrading, and downgrading of dependencies should be done using 'go get'. See 'go help modules' for an overview of module functionality. Usage: go mod <command> [arguments] The commands are: download download modules to local cache 修改 go.mod 文件后,下载更新的依赖模块 edit edit go.mod from tools or scripts graph print module requirement graph init initialize new module in current directory tidy add missing and remove unused modules 移除掉 go.mod 中没被使用的 require 模块 vendor make vendored copy of dependencies verify verify dependencies have expected content why explain why packages or modules are needed Use "go help mod <command>" for more information about a command.
7. Go Playground
Go 官方提供了一个在线运行、分享 Go 语言代码的平台 Go Playground
,国内访问不了,感兴趣的可以根据 https://github.com/golang/playground 自行搭建
8. 入门必读
A Tour of Go
是 Go 官方出品的交互式教程,包含了 Go 编程基础知识的许多示例程序,浅显易懂。如果英语水平可以的话建议直接看原版英文的,感觉中文翻译的有些地方不准确
Effective Go
教你如何写出优雅的 Go 风格代码:
- 英文版: https://golang.org/doc/effective_go.html 或 https://golang.google.cn/doc/effective_go.html
- 中文版: https://go-zh.org/doc/effective_go.html
Golang 规范: https://golang.google.cn/ref/spec
Golang 命令文档: https://golang.google.cn/cmd/go/
Golang wiki: https://github.com/golang/go/wiki
Golang 内存模型: https://golang.google.cn/ref/mem
Golang 诊断: https://golang.google.cn/doc/diagnostics
分类: Go Basic
标签: golang.org/x Goland GOPROXY Go Modules
分享
相关推荐
作者
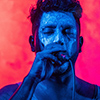
0 条评论
评论者的用户名
评论时间暂时还没有评论.